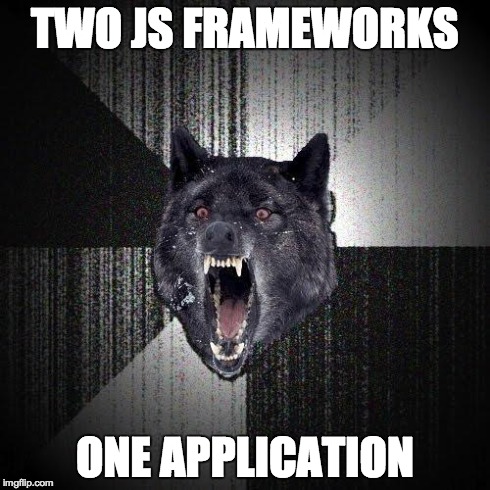
Who's this dude?
- Gordon Hempton
- Works at outreach.io
- Been using Ember for a long time
- Author of EmberScript and EPF
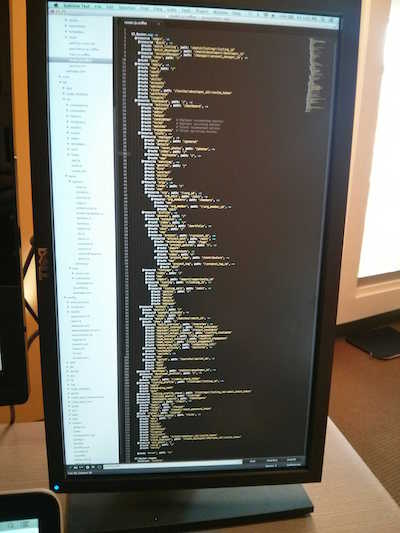
Two Frameworks? Are you Crazy?
Ember + Ember-Data + jQuery + Handlebars ~= 811k (minified)
React ~= 126k (minified)
Why Switch To React?
Worst framework performance comparison ever:
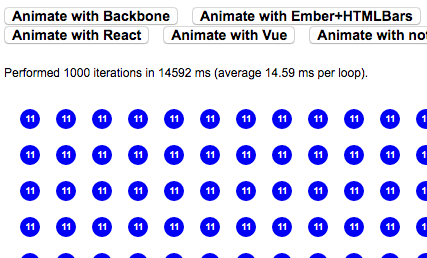
. @floydophone Ember.js will be the #1 JS framework for spinning circles
— Erik Bryn (@ebryn) January 17, 2014
Initial Render is Our Bottleneck
Ember is heavily optimized for changes, not the initial render
Our App
outreach.io
Perf Optimization Sucks
Some solutions:
- {{group}}
- Ember.ListView
- Ember Table
- Custom render() method (what we use)
What we want:
- Performance optimizations should be few and far between
- Still benefit from our framework choice in hot code paths
- Reuse as much code as possible
Demo
React components
// file: app/react/time-ago.js
/** @jsx React.DOM */
export default React.createClass({
render: function() {
var date = this.props.date;
return (
<time datetime={date}>{moment(date).fromNow()}</time>;
);
}
});
Inside your Ember templates
<div>{{react 'time-ago' date=date}}<div>
React Router
Based on the Ember.js Router :)
React Routing
<Routes location="history">
<Route name="profiles"
path={rootPath + '/profiles'}
handler={ProfilesIndex} />
<Route name="routing"
path={rootPath + '/routing'}
handler={Routing} />
</Routes>
Inside your Ember Routes
export default Ember.Route.extend({
renderTemplate: function() {
var rootPath = this.router.generate(this.routeName);
this.render({
react: ...
});
}
});
Inside the Ember Router DSL
Router.map(function() {
this.react('posts');
});
Observations
- Ember.js is really good at reacting to incremental changes
- React is really good at rendering everything
- React comes with its own set of opinions (Immutability anyone?)
- React is slightly awkward with complex models
- Ember conventions can be used outside of Ember
When to Use React within an existing Ember app?
- Performance
- Composability
- Complex Rendering
- Masochism
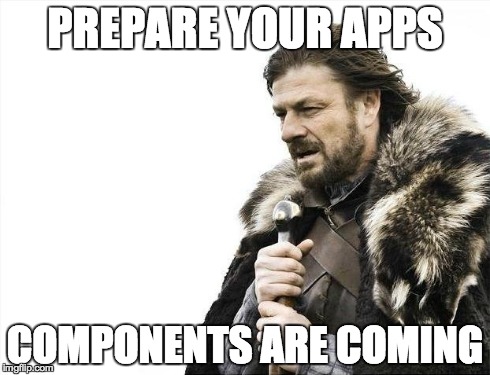
Both Angular 2.0 and Ember 2.0 look a lot like React
From the Ember 2.0 RFC:
In Ember 2.0, we will be adopting a "virtual DOM" and data flow model that embraces the best ideas from React and simplifies communication between components.
We plan to transition to: when a route is entered, it renders a component, passing along the model as an attr. This eliminates a vestigial use of old-style views, and associates the top-level template with a regular component.
Ember 2.0 | Our React Stack |
---|---|
|
|